A binary search tree (BST) is a node based binary tree data structure which has the following properties.
• The left subtree of a node contains only nodes with keys less than the node’s key.
• The right subtree of a node contains only nodes with keys greater than the node’s key.
• Both the left and right subtrees must also be binary search trees.
From the above properties it naturally follows that:
• Each node (item in the tree) has a distinct key.
Solution:
- Start from root with min = -INF and max = + INF
- At each node check whether node's data is in range [min,max]
- If not it violates binary search tree,return 0.
- you can use the below isbst() trace,
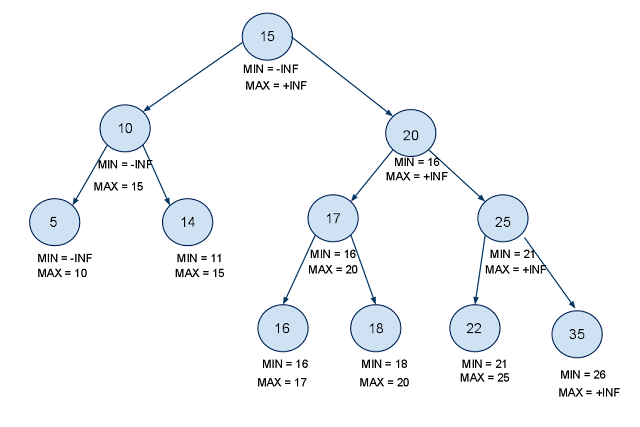
Complexity: worst case - when it is a bst, O(n) where n is the number of nodes.
Code:
int isBSTUtil(Tnode *root,int min,int max) { if(root==NULL) return 1; if(root->data < min || root->data >= max) return 0; return isBSTUtil(root->left,min,root->data) && isBSTUtil(root->right, root->data + 1, max); } int isBST(Tnode *root) { int min=1<<((sizeof(int)*8)-1); int max=~min; //printf("min=%d max=%d",min,max); return isBSTUtil(root,min,max); }
No comments:
Post a Comment